作为补充笔记(未完工),有些自己组织的语言可能错误,内容还是以课本为主
What Are Algorithms and Why Should You Care?
How to Describe and Evaluate Computer Algorithms
Algorithms are a fundamental part of computer programs. Whether you are multiplying two numbers or processing images sent by spacecrafts billions of mile away, you need algorithms to solve the problem at hand correctly and efficiently.
算法在我们的生活处处可见,我们研究算法,是为了高效而又正确的解决问题,通过学习算法提高思维能力...
这里的效率有多方面的考量,占用的空间资源,运算的时间长度
Linear Search
通过顺序查找所需要的值,我们还要保证严密,在未找到数据时候返回信息,不能单认为所求必在其中
How to characterize running times
If you know a bit about actual computer architecture, you might know that the time to access a given variable or array element is not necessarily fixed, for it could depend on whether the variable or array element is in the cache, in main memory, or out on disk in a virtual-memory system.
在计算机中,进行某个步骤都要一定的时间,通过求和,我们能得到关系式(包含上下界,一般对应最坏以及最好情况)
书中通过时间复杂度进行概括,不能单纯理解为使用的seconds,应该理解为运算的步骤数的数量级,为什么?不同的配置的硬件对运算的时长影响也很大,我们通过关注算法运算时间的增长量级(在较大数量级下得到结果的步骤数进行抽象)得到时间复杂度的概念
渐进符号
\Theta符号
\Theta{(g(n))=\{f(n)}:存在两个正数{c_1,c_2}和{n_0}使得{0\le c_1g(n)\le f(n)\le c_2g(n),n>=n_0}\}{g(n)}是f(n)的渐进紧确界(asymptotically tight bound),
O符号
渐进上界 最坏时间
O{(g(n))=\{f(n)}:在正数{c}和{n_0}得{0\le f(n)\le cg(n),n>=n_0}\}
\Omega符号
渐进下界 最优时间
\Omega{(g(n))=\{f(n)}:在正数{c}和{n_0}得{0\le cg(n)\le f(n),n>=n_0}\}
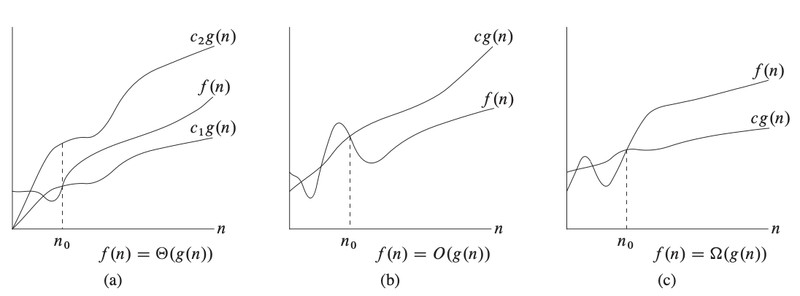
o符号
无穷小量
o{(g(n))=\{f(n)}:对于任意正数{c},存在{n_0}得{0\le f(n)< cg(n),n>=n_0}\}
\omega符号
无穷大量
\omega{(g(n))=\{f(n)}:对于任意正数{c},存在{n_0}得{0\le cg(n)< f(n),n>=n_0}\}
PS:有时间复杂度也有空间复杂度,暂时不提及
Loop Invariants(循环不变式)
A loop invariant is a tool used for proving statements about the properties of our algorithms and programs.
Naturally, correctness is the property we’re most interested in.
We should be sure that our algorithms always produce correct results before putting them to use.
在计算机执行一个有迭代、循环的算法时,我们很难跟踪其中发生了什么(除非使用调试等技巧),很多时候会遇到未达到目标的情况下循环不终止或者提前终止的行为
因此,我们引出了Loop Invariants,它能够正确说明程序中变量的关系,在执行前为真(Initialize),执行过程也为真(Maintenance)
Termination又怎么理解
The test condition of the loop is not part of the invariant. It is what makes the loop terminate.
The loop will terminate if each time through the loop you move closer to satisfying the termination condition.
与数学归纳法很相似,但是数学归纳法多研究无穷的情况,Loop Invariants多研究数据集范围内的情况
Recursion
称作递归,需要base case才能使得其正常返回
Algorithms for Sorting and Searching
Binary Search
和高中所学的几乎没有差异,通过中位数分半缩小探查范围
Selection Sort
Insertion Sort
两者很相似,放一个插入排序的伪代码
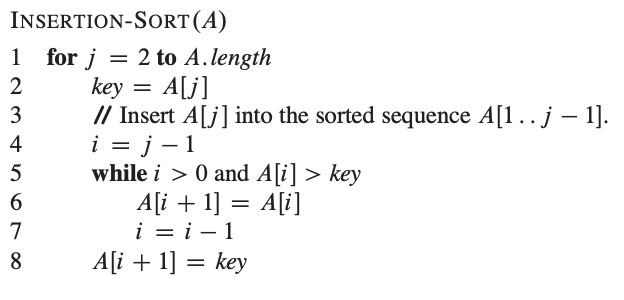
Merge Sort
Quick Sort
将问题分而治之的排序算法
分治思想:
- 分划为子问题
- 递归的攻破问题
- 结合子问题的答案形成完整解
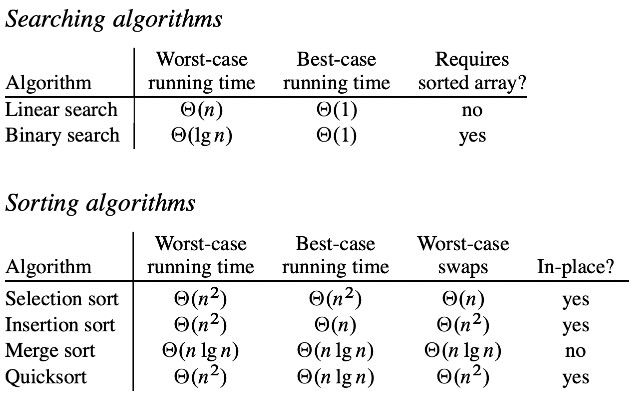
上述算法最好的平均时间复杂度为\Theta(nlgn),因为他们都是少不了元素间的比较,他们会生成一棵元素两两比较的决策树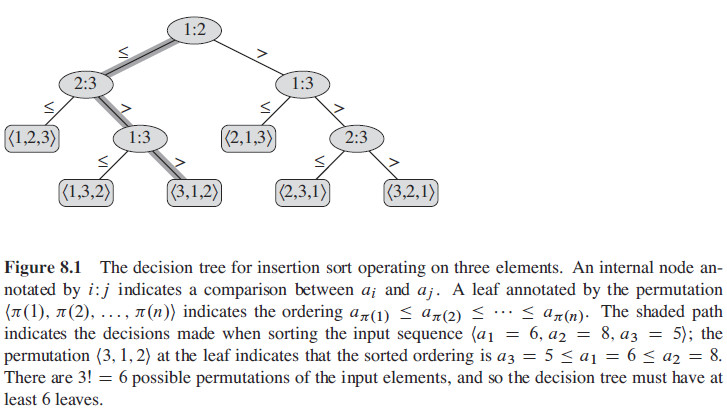
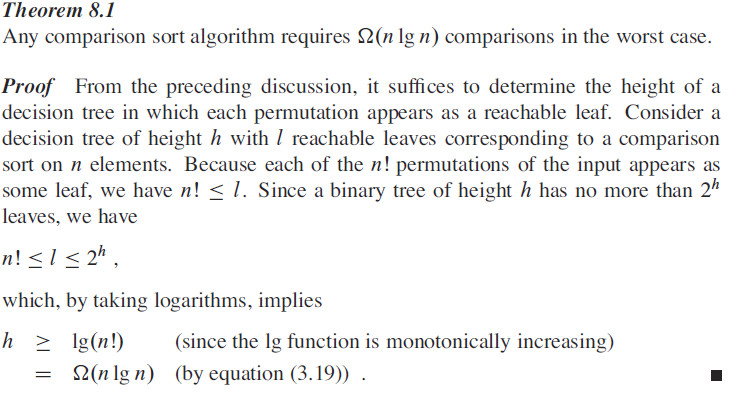
当然也存在一些线性时间的排序算法\Theta(n)
计数排序、基数排序、桶排序...